Real pro’s debug with console.log
;-)
So let’s build a the world’s smallest logging library. It is called picolog or plog
for short as has ZERO dependencies.
// picolog.js
export default function plog ( ctx, out = console.log ) {
return ctx ? (...args) => out('[' + ctx + ']', ...args) : () => {};
}
import plog from '@picolog'
const log = plog('foobar'); // create a logger with context 'foobar'
const log = plog(false); // create a disabled logger
const log = plog(!'foobar'); // ...or disable logger by adding '!'
const log = plog('foobar', () => {}); // ...or by passing an empty function
const error = plog('foobar', console.error); // create a logger that logs to console.error
now start logging with:
log('hello', 'world', 42)
or get fancy with:
import plog from '@picolog'
const fancy_out = (...args) => {
console.log("🤖 %c" + args[0],
"color: white; background-color: #69b42d",
...args.slice(1));
}
const log = plog('context', fancy_out); // create a logger with fance output
log("hello world", 0)
log("hello world", 1)
...
Combining this with the filter function of the browser dev tools is actually pretty useful. Just filter by context
.
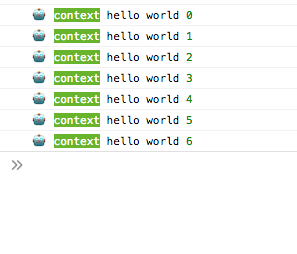